Signup
Let's cut to the chase. Here's an example of a Marcellus Wallace signing up. Details will be discussed in the following sections.
Load SubscriptionJS and initialize basic objects. For identification you need to provide your publicApiKey
. The providerReturnUrl
specifies a page on your website a customer returns to after payment on a payment provider page (e.g. PayPal).
<script type="text/javascript" src="https://selfservice.sandbox.billwerk.com/subscription.js"></script> <script type="text/javascript"> var signupService = new SubscriptionJS.Signup(); var paymentService = new SubscriptionJS.Payment({ publicApiKey : "527cc4c951f45909c493c820", providerReturnUrl : "https://your_domain.com/your_finalize_page" }, function () { /*Everything initialized so we can go on... Start processing order, because example does not have a form. In real life you would probably set a flag that initialization is finished.*/ createOrder(); }, function() { /*error*/ }); // Important! SubscriptionJS.Payment is not necessarily ready here. Use success callback to go on in order process </script>
Important
Important! SubscriptionJS.Payment does asynchronous initialization of the PSP specific JS code. Do not start order process until success callback is called!
The following section is hard coded data that would usually be entered by the customer within a form. This is just for simplicity of the example.
var cart = { "planVariantId": "527caacdeb596a247c6e0500" }; var customer = { "firstName": "Marcellus", "lastName": "Wallace", "emailAddress": "mhw@example.com" }; var paymentData = { "bearer": "CreditCard:Paymill", "cardNumber": "5169147129584558", "expiryMonth": "12", "expiryYear": "2017", "cardHolder": "Marcellus Wallace", "cvc": "311" };
Step 1: Create the order
The first step in the subscription process is creating an order. The function createOrder(...)
takes the cart
and customer
data, as well as a success and error callback.
//Step 1: Create order var createOrder = function() { signupService.createOrder(cart, customer, pay, //Order created. Go on... errorHandler //Order could not be created ); }
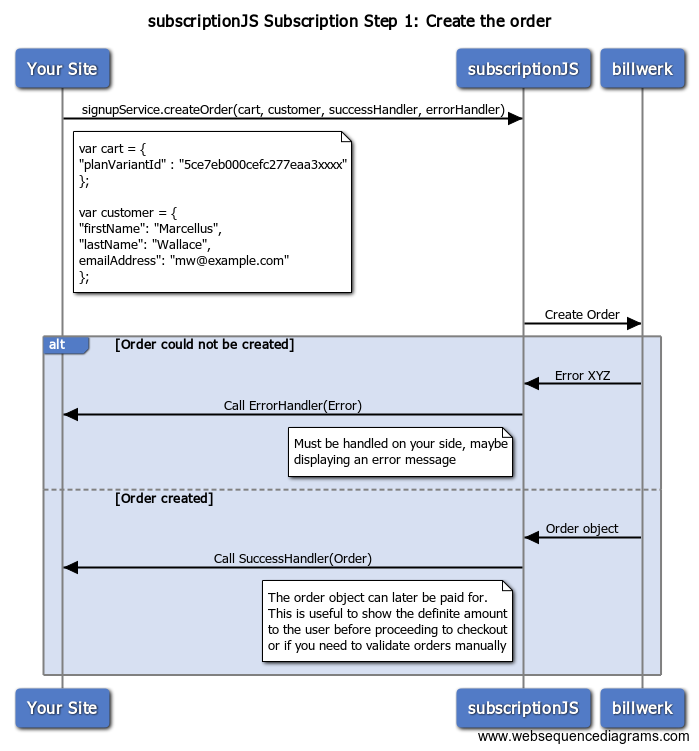
After successful creation of the order the payment process is triggered. The function paySignupInteractive(...)
takes paymentService
, paymentData
and the order
that was returned in the last step. You also pass a success and error callback. This step is also done for free subscriptions. It'll simply finish the order process without triggering any payment.
Notice
In reality, the integration step 1 (order creation) and 2 (order commit / payment) should be implemented on separate pages to be able to handle the order process within the context of a single order.
Step 2: Commit the order
//Step 2: Trigger payment / order commit var pay = function(order) { signupService.paySignupInteractive(paymentService, paymentData, order, paymentHandler, //Everything ok so far. Go on... errorHandler //Payment failed ); };
If step 2 returned successfully there are two possible use cases:
The payment could already be finished without a visible payment provider page.
The payment process requires the customer to be forwarded to the payment provider page (e.g. PayPal checkout). After the payment PSPs checkout page the customer will be forwarded to the Url you specified during initialization in
providerReturnUrl
.//Step 3: Forward to PSP page if not already finished var paymentHandler = function (result) { if (!result.Url) alert("success!"); //Successful subscription else window.location.href = result.Url; //Forward to PSP page to pay }
Caution
Caution! Special case for PayEx CreditCard! In case you are using our paymentForm the input fields for Card Holder, Credit Card Number, CVC and ExpiryDate are not displayed.
Only the selected logos inside the PayEx settings are displayed. paySignupInteractive will redirect your customer to the PayEx CreditCard Checkout Form, where the input fields Card Holder, Credit Card Number, CVC and ExpiryDate are displayed.
Caution
Caution! Special requirements for Unzer. See Unzer FAQ.
The rest of the example is a very simple error handler and a call executing the example. In a real world error handler you'd probably do handling based on the error code returned, e.g. let the user change credit card number if it was invalid. You can call
paySignupInteractive
several times if previous payments failed. Billwerk will take care that only a single successful payment is processed for an order.Notice
Beware the user from double clicking the order button. billwerk will still handle the payments correctly, but it would most probably produce undesired effects in your order process.
var errorHandler = function(errorData) { alert("Subscription failed"); }; createOrder();
Step 3: Finalize the order
As shown in the last examples some payment provider integrations involve redirecting to the PSP's checkout page. The PSP will forward the user to the Url you provided in a providerReturnUrl
after the customer has finished checkout. This Url is called the finalize page. It is the last page of the order process.
On this page you need to call the SubscriptionJS function finalize(...)
. The data structure passed to the functions is the same as in step 3 of the initial example.
SubscriptionJS.finalize(success,error);
Notice
Don't truncate the Url parameters used by the PSP. SubscriptionJS needs this information.
The simple approach (Combination of the Step 1+2)
SubscriptionJS also provides a way to combine step 1 and 2 of the initial example. In this case you just need a single call, a success and an error handler.
signupService.subscribe(paymentService, cart, customer, paymentData, function (subscribeResult) { if (!result.Url) alert("success!"); //Successful subscription else window.location.href = result.Url; //Forward to PSP page to pay }, function (errorData) { alert("something went wrong!"); } );
Its simplicity may be tempting, but combining order creation and payment in a single step can lead to serious problems if not handled correctly. Customers could easily end up placing two or more orders by going back in the order process and clicking the order button a second or third time.
Each click will produce a separate order and payment. You also can't offer a convenient way to change payment data, if the customer made a mistake. We strongly encourage you to use the approach with separated order creation and order commit / payment you saw in the first example.
Notice
Don't use the simple approach if the preferred approach of separated steps is applicable for you